Adding GIF image in an ImageView in android
use android-gif-drawable library ๐
add the following dependency to build.gradle
file of your project.
dependencies {
implementation 'pl.droidsonroids.gif:android-gif-drawable:1.2.19'
}
Use the view in XML file like this.
<pl.droidsonroids.gif.GifImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/src_anim"
android:background="@drawable/bg_anim"
/>
For more customization and code, see the docs here .
use VideoView on Android ๐
You can use VideoView
to show the GIF image. But the library is more efficient and smooth.
use ImageView on Android ๐
Use ImageView and Split the GIF file into several images and then apply animation to it. It is complicated and not worth it because the library is more efficient and elegant.
use Glide library ๐
add the dependency to build.gradle
like this.
repositories {
mavenCentral()
google()
}
dependencies {
implementation 'com.github.bumptech.glide:glide:4.8.0'
annotationProcessor 'com.github.bumptech.glide:compiler:4.8.0'
}
Then in activity or fragment use this code.
ImageView imageView = findViewById(R.id.imageView);
If you want to pull the image from the Internet, use this code snippet.
Glide.with(this)
.load("https://media.giphy.com/media/98uBZTzlXMhkk/giphy.gif")
.into(imageView);
But if you want to use a local image from raw folder in your Android app, use this code snippet.
Glide.with(this)
.load(R.raw.giphy)
.into(imageView);
If you are already using Glide, use the code above. But if you are not using Glide in your app, just use the first library because it is lightweight and efficient.
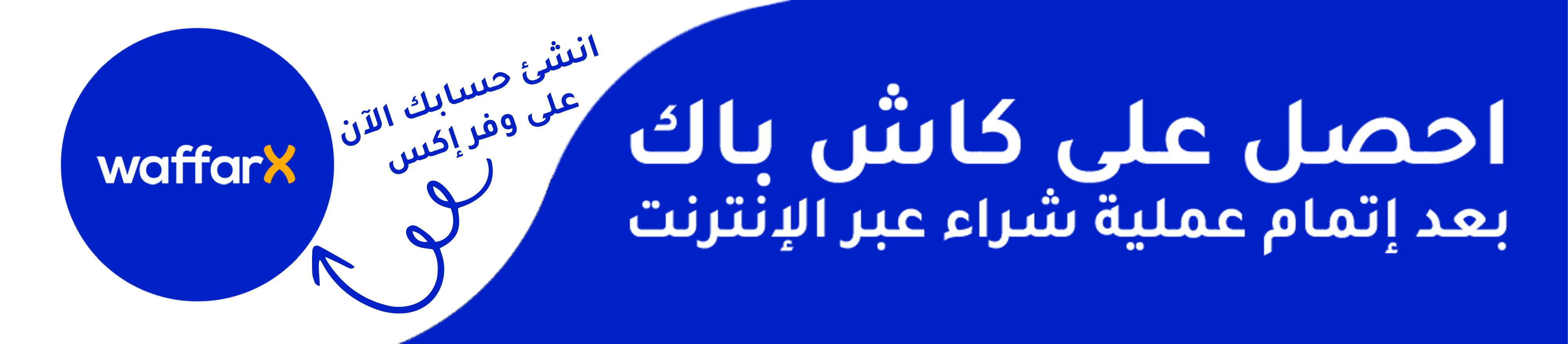