How do you animate the change of background color of a view on Android ?
·
211 words
·
1 minute read
The idea is to use this code snippet.
ColorDrawable[] colorDrawables = {
new ColorDrawable(Color.RED),
new ColorDrawable(Color.BLUE),
new ColorDrawable(Color.GREEN)
};
TransitionDrawable transitionDrawable = new TransitionDrawable(colorDrawables);
textView.setBackground(transitionDrawable);
transitionDrawable.startTransition(2000);
In this code snippet, we created a list of colorDrawables, then feed them to the TransitionDrawable. And now we have an animating color change, so we pass it to the setBackground
to get the animating colors in the background. Got the idea ? let’s create a complete code example.
- use this xml code in
res/layout/activity_main.xml
.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/relativeLayout"
android:padding="8dp"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:text="Animate background color"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@id/textView"
android:layout_centerInParent="true"
android:layout_marginBottom="15dp"/>
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="36sp"
android:textStyle="bold"
android:text="Changing Background color of this view."
android:layout_centerInParent="true" />
</RelativeLayout>
- use this [Kotlin] code in
src/MainActivity.java
file.
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Color;
import android.graphics.drawable.ColorDrawable;
import android.graphics.drawable.TransitionDrawable;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
TextView textView;
Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button = findViewById(R.id.button);
textView = findViewById(R.id.textView);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
ColorDrawable[] colorDrawables = {new ColorDrawable(Color.RED),
new ColorDrawable(Color.BLUE), new ColorDrawable(Color.GREEN)};
TransitionDrawable transitionDrawable = new TransitionDrawable(colorDrawables);
textView.setBackground(transitionDrawable);
transitionDrawable.startTransition(2000);
}
});
}
}
Run the application. You’ll see the background of the textview is animating when you touch / tap it.
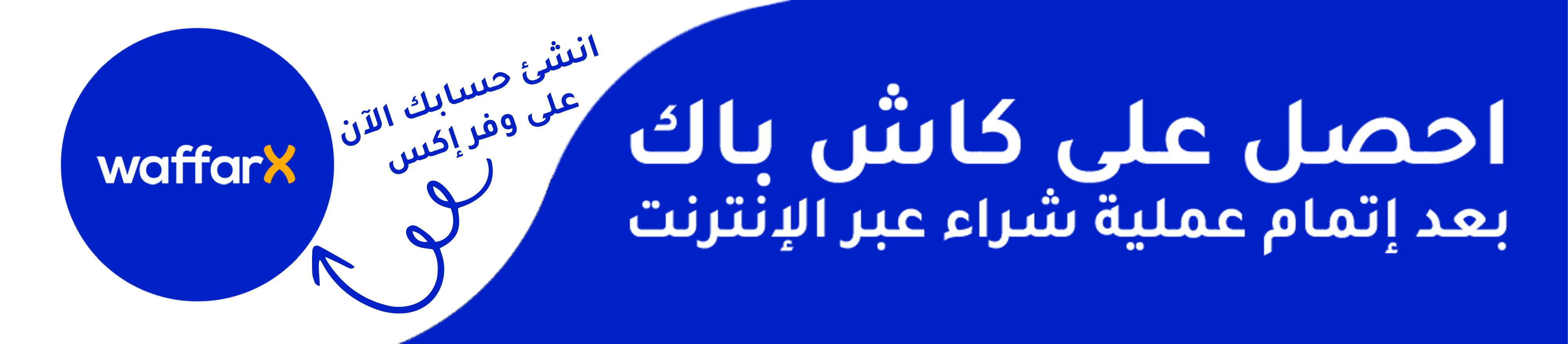