How to Change Listview Item Color in Android ?
·
237 words
·
2 minute read
The idea is to create an a list item xml, and give it the design choices you want. If you want to change the list item color, use this xml code android:textColor="@android:color/holo_orange_dark"
.
If you want to change the text to italic or bold, use this xml android:textStyle="italic|bold"
. And even if you want to change the font itself, you can by this font-family property in xml android:fontFamily="sans-serif-condensed"
.
Now, you got the idea. Let’s build a sample application to illustrate this more practically.
- create Android app in Android Studio
- use this XML as a
res/layout/activity_main.xml
.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:textColor="@android:color/holo_orange_dark"
android:textSize="24sp"
android:textStyle="italic|bold" />
</LinearLayout>
- use this Kotlin code in your
src/MainActivity.kt
.
import android.os.Bundle
import android.widget.ArrayAdapter
import android.widget.ListView
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
var operatingSystem: Array<String> = arrayOf("Android", "IPhone", "WindowsMobile", "Blackberry", "WebOS", "Ubuntu", "Windows7", "Max OS X")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
title = "KotlinApp"
val listView: ListView = findViewById(R.id.listView)
val adapter = object : ArrayAdapter<String>(this, R.layout.list_item, R.id.textView, operatingSystem) {
}
listView.adapter = adapter
}
}
- create a layout resource file, and name it
list_item.xml
. It represents an item in the listview.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:textColor="@android:color/holo_orange_dark"
android:textSize="24sp"
android:textStyle="italic|bold" />
</LinearLayout>
Run the application, and you’ll see the listview items is configurable via list_item.xml
file. Change the color of the item as you want, and re-run the app.
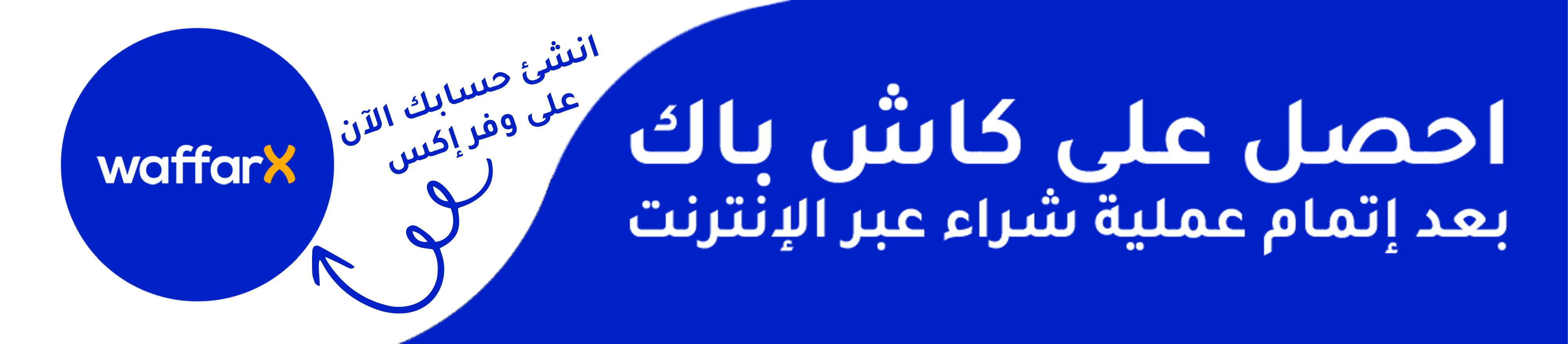