How to Draw Rectangle on Imageview ?
If you already have a bitmap image in your code, just use this code snippet.
bmp = bmp.copy(Bitmap.Config.RGB_565, true);
That code will recreate the bitmap image as a mutable. So you can edit that bitmap image on canvas as you like.
But if you do not have a bitmap image in code, create a new one using this code snippet.
Bitmap bmp=Bitmap.createBitmap(img.getHeight(),img.getWidth(),Bitmap.Config.RGB_565);
where img
is another bitmap image you want to make a new one with the height and width of it. If you want to create one from scratch here is the code snippet.
Bitmap bmp=Bitmap.createBitmap(400,700,Bitmap.Config.RGB_565);
After that, create a canvas and pass the bitmap image you have.
Canvas canvas = new Canvas(bmp);
If you want to draw the bitmap image on the canvas after creating the canvas, use this code snippet.
Canvas canvas = new Canvas();
// bitmap from path
canvas.drawBitmap(BitmapFactory.decodeFile(picPath), 0, 0, null);
// bitmap itself
canvas.drawBitmap(btmp, 0, 0, null);
Then, choose the painting options for the rectangles as you can see in the following code snippet.
Paint paint = new Paint();
paint.setAlpha(0xA0); // the transparency
paint.setColor(Color.RED); // color is red
paint.setStyle(Paint.Style.STROKE); // stroke or fill or ...
paint.setStrokeWidth(1); // the stroke width
After choosing the painting options, write the dimensions of the rectangles using this code.
Rect r = new Rect(0, 0, 10, 20);
The rectangle will be draw / painted from point (0,0) to point (10,20).
To draw that rectangle on the canvas, use this code snippet.
canvas.drawRect(r, paint);
After finish editing the canvas, re-assign the new bitmap (edited with canvas) to the imageview using this code snippet in [Java].
imageView.setImageBitmap(bmp);
That code will make sure the changes appear on the bitmap of imageview which the user can see.
WE’RE DONE; I hope this post helps you. Do you recommend reading this blog post ? share it !
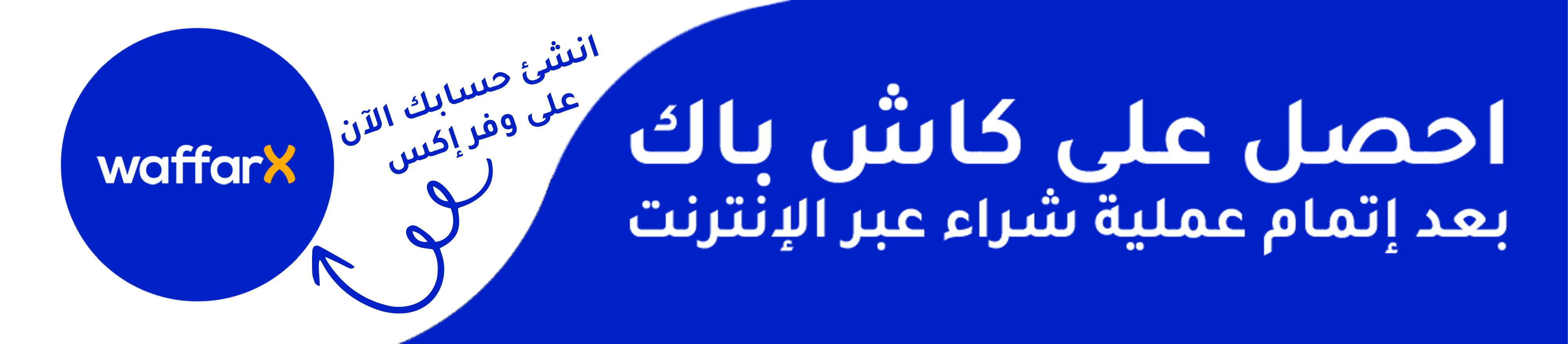