Git Cheatsheet | Distributed Version Control System
git π
Distributed version control system.
Execute a Git subcommand:
git subcommand
Execute a Git subcommand on a custom repository root path:
git -C path/to/repo subcommand
Execute a Git subcommand with a given configuration set:
git -c 'config.key=value' subcommand
Display help:
git --help
Display help for a specific subcommand (like clone
, add
, push
, log
, etc.):
git help subcommand
Display version:
git --version
git submodule π
Inspects, updates and manages submodules.
Install a repository’s specified submodules:
git submodule update --init --recursive
Add a Git repository as a submodule:
git submodule add repository_url
Add a Git repository as a submodule at the specified directory:
git submodule add repository_url path/to/directory
Update every submodule to its latest commit:
git submodule foreach git pull
git pull π
Fetch branch from a remote repository and merge it to local repository.
Download changes from default remote repository and merge it:
git pull
Download changes from default remote repository and use fast-forward:
git pull --rebase
Download changes from given remote repository and branch, then merge them into HEAD:
git pull remote_name branch
git reset π
Undo commits or unstage changes, by resetting the current Git HEAD to the specified state.
If a path is passed, it works as “unstage”; if a commit hash or branch is passed, it works as “uncommit”.
Unstage everything:
git reset
Unstage specific file(s):
git reset path/to/file1 path/to/file2 ...
Interactively unstage portions of a file:
git reset --patch path/to/file
Undo the last commit, keeping its changes (and any further uncommitted changes) in the filesystem:
git reset HEAD~
Undo the last two commits, adding their changes to the index, i.e. staged for commit:
git reset --soft HEAD~2
Discard any uncommitted changes, staged or not (for only unstaged changes, use git checkout ):
git reset --hard
Reset the repository to a given commit, discarding committed, staged and uncommitted changes since then:
git reset --hard commit
git bugreport π
Captures debug information from the system and user, generating a text file to aid in the reporting of a bug in Git.
Create a new bug report file in the current directory:
git bugreport
Create a new bug report file in the specified directory, creating it if it does not exist:
git bugreport --output-directory path/to/directory
Create a new bug report file with the specified filename suffix in strftime
format:
git bugreport --suffix %m%d%y
git gc π
Optimise the local repository by cleaning unnecessary files.
Optimise the repository:
git gc
Aggressively optimise, takes more time:
git gc --aggressive
Do not prune loose objects (prunes by default):
git gc --no-prune
Suppress all output:
git gc --quiet
Display help:
git gc --help
git diff-files π
Compare files using their sha1 hashes and modes.
Compare all changed files:
git diff-files
Compare only specified files:
git diff-files path/to/file
Show only the names of changed files:
git diff-files --name-only
Output a summary of extended header information:
git diff-files --summary
git symbolic-ref π
Read, change, or delete files that store references.
Store a reference by a name:
git symbolic-ref refs/name ref
Store a reference by name, including a message with a reason for the update:
git symbolic-ref -m "message" refs/name refs/heads/branch_name
Read a reference by name:
git symbolic-ref refs/name
Delete a reference by name:
git symbolic-ref --delete refs/name
For scripting, hide errors with --quiet
and use --short
to simplify (“refs/heads/X” prints as “X”):
git symbolic-ref --quiet --short refs/name
git update-ref π
Git command for creating, updating, and deleting Git refs.
Delete a ref, useful for soft resetting the first commit:
git update-ref -d HEAD
Update ref with a message:
git update-ref -m message HEAD 4e95e05
git var π
Prints a Git logical variable’s value.
See git config
, which is preferred over git var
.
Print the value of a Git logical variable:
git var GIT_AUTHOR_IDENT|GIT_COMMITTER_IDENT|GIT_EDITOR|GIT_PAGER
[l]ist all Git logical variables:
git var -l
git range-diff π
Compare two commit ranges (e.g. two versions of a branch).
Diff the changes of two individual commits:
git range-diff commit_1^! commit_2^!
Diff the changes of ours and theirs from their common ancestor, e.g. after an interactive rebase:
git range-diff theirs...ours
Diff the changes of two commit ranges, e.g. to check whether conflicts have been resolved appropriately when rebasing commits from base1
to base2
:
git range-diff base1..rev1 base2..rev2
git stash π
Stash local Git changes in a temporary area.
Stash current changes, except new (untracked) files:
git stash push -m optional_stash_message
Stash current changes, including new (untracked) files:
git stash -u
Interactively select parts of changed files for stashing:
git stash -p
List all stashes (shows stash name, related branch and message):
git stash list
Show the changes as a patch between the stash (default is stash@{0}
) and the commit back when stash entry was first created:
git stash show -p stash@{0}
Apply a stash (default is the latest, named stash@{0}
):
git stash apply optional_stash_name_or_commit
Drop or apply a stash (default is stash@{0}
) and remove it from the stash list if applying doesn’t cause conflicts:
git stash pop optional_stash_name
Drop all stashes:
git stash clear
git count-objects π
Count the number of unpacked objects and their disk consumption.
Count all objects and display the total disk usage:
git count-objects
Display a count of all objects and their total disk usage, displaying sizes in human-readable units:
git count-objects --human-readable
Display more verbose information:
git count-objects --verbose
Display more verbose information, displaying sizes in human-readable units:
git count-objects --human-readable --verbose
git rev-list π
List revisions (commits) in reverse chronological order.
List all commits on the current branch:
git rev-list HEAD
Print the latest commit that changed (add/edit/remove) a specific file on the current branch:
git rev-list -n 1 HEAD -- path/to/file
List commits more recent than a specific date, on a specific branch:
git rev-list --since='2019-12-01 00:00:00' branch_name
List all merge commits on a specific commit:
git rev-list --merges commit
Print the number of commits since a specific tag:
git rev-list tag_name..HEAD --count
git show π
Show various types of Git objects (commits, tags, etc.).
Show information about the latest commit (hash, message, changes, and other metadata):
git show
Show information about a given commit:
git show commit
Show information about the commit associated with a given tag:
git show tag
Show information about the 3rd commit from the HEAD of a branch:
git show branch~3
Show a commit’s message in a single line, suppressing the diff output:
git show --oneline -s commit
Show only statistics (added/removed characters) about the changed files:
git show --stat commit
Show only the list of added, renamed or deleted files:
git show --summary commit
Show the contents of a file as it was at a given revision (e.g. branch, tag or commit):
git show revision:path/to/file
git checkout π
Checkout a branch or paths to the working tree.
Create and switch to a new branch:
git checkout -b branch_name
Create and switch to a new branch based on a specific reference (branch, remote/branch, tag are examples of valid references):
git checkout -b branch_name reference
Switch to an existing local branch:
git checkout branch_name
Switch to the previously checked out branch:
git checkout -
Switch to an existing remote branch:
git checkout --track remote_name/branch_name
Discard all unstaged changes in the current directory (see git reset for more undo-like commands):
git checkout .
Discard unstaged changes to a given file:
git checkout path/to/file
Replace a file in the current directory with the version of it committed in a given branch:
git checkout branch_name -- path/to/file
git commit-graph π
Write and verify Git commit-graph files.
Write a commit-graph file for the packed commits in the repository’s local .git
directory:
git commit-graph write
Write a commit-graph file containing all reachable commits:
git show-ref --hash | git commit-graph write --stdin-commits
Write a commit-graph file containing all commits in the current commit-graph file along with those reachable from HEAD
:
git rev-parse HEAD | git commit-graph write --stdin-commits --append
git difftool π
Show file changes using external diff tools. Accepts the same options and arguments as git diff .
List available diff tools:
git difftool --tool-help
Set the default diff tool to meld:
git config --global diff.tool "meld"
Use the default diff tool to show staged changes:
git difftool --staged
Use a specific tool (opendiff) to show changes since a given commit:
git difftool --tool=opendiff commit
git rev-parse π
Display metadata related to revisions.
Get the commit hash of a branch:
git rev-parse branch_name
Get the current branch name:
git rev-parse --abbrev-ref HEAD
Get the absolute path to the root directory:
git rev-parse --show-toplevel
git repack π
Pack unpacked objects in a Git repository.
Pack unpacked objects in the current directory:
git repack
Also remove redundant objects after packing:
git repack -d
git credential-store π
git
helper to store passwords on disk.
Store Git credentials in a specific file:
git config credential.helper 'store --file=path/to/file'
git daemon π
A really simple server for Git repositories.
Launch a Git daemon with a whitelisted set of directories:
git daemon --export-all path/to/directory1 path/to/directory2
Launch a Git daemon with a specific base directory and allow pulling from all sub-directories that look like Git repositories:
git daemon --base-path=path/to/directory --export-all --reuseaddr
Launch a Git daemon for the specified directory, verbosely printing log messages and allowing Git clients to write to it:
git daemon path/to/directory --enable=receive-pack --informative-errors --verbose
git column π
Display data in columns.
Format stdin
as multiple columns:
ls | git column --mode=column
Format stdin
as multiple columns with a maximum width of 100
:
ls | git column --mode=column --width=100
Format stdin
as multiple columns with a maximum padding of 30
:
ls | git column --mode=column --padding=30
git worktree π
Manage multiple working trees attached to the same repository.
Create a new directory with the specified branch checked out into it:
git worktree add path/to/directory branch
Create a new directory with a new branch checked out into it:
git worktree add path/to/directory -b new_branch
List all the working directories attached to this repository:
git worktree list
Remove a worktree (after deleting worktree directory):
git worktree prune
git cherry-pick π
Apply the changes introduced by existing commits to the current branch.
To apply changes to another branch, first use git checkout to switch to the desired branch.
Apply a commit to the current branch:
git cherry-pick commit
Apply a range of commits to the current branch (see also git rebase --onto
):
git cherry-pick start_commit~..end_commit
Apply multiple (non-sequential) commits to the current branch:
git cherry-pick commit1 commit2 ...
Add the changes of a commit to the working directory, without creating a commit:
git cherry-pick --no-commit commit
git notes π
Add or inspect object notes.
List all notes and the objects they are attached to:
git notes list
List all notes attached to a given object (defaults to HEAD):
git notes list [object]
Show the notes attached to a given object (defaults to HEAD):
git notes show [object]
Append a note to a specified object (opens the default text editor):
git notes append object
Append a note to a specified object, specifying the message:
git notes append --message="message_text"
Edit an existing note (defaults to HEAD):
git notes edit [object]
Copy a note from one object to another:
git notes copy source_object target_object
Remove all the notes added to a specified object:
git notes remove object
git ls-tree π
List the contents of a tree object.
List the contents of the tree on a branch:
git ls-tree branch_name
List the contents of the tree on a commit, recursing into subtrees:
git ls-tree -r commit_hash
List only the filenames of the tree on a commit:
git ls-tree --name-only commit_hash
Print the filenames of the current branch head in a tree structure (Note: tree --fromfile
is not supported on Windows):
git ls-tree -r --name-only HEAD | tree --fromfile
git shortlog π
Summarizes the git log
output.
View a summary of all the commits made, grouped alphabetically by author name:
git shortlog
View a summary of all the commits made, sorted by the number of commits made:
git shortlog -n
View a summary of all the commits made, grouped by the committer identities (name and email):
git shortlog -c
View a summary of the last 5 commits (i.e. specify a revision range):
git shortlog HEAD~5..HEAD
View all users, emails and the number of commits in the current branch:
git shortlog -sne
View all users, emails and the number of commits in all branches:
git shortlog -sne --all
git diff-index π
Compare the working directory with a commit or tree object.
Compare the working directory with a specific commit:
git diff-index commit
Compare a specific file or directory in working directory with a commit:
git diff-index commit path/to/file_or_directory
Compare the working directory with the index (staging area) to check for staged changes:
git diff-index --cached commit
Suppress output and return an exit status to check for differences:
git diff-index --quiet commit
git status π
Show the changes to files in a Git repository.
Lists changed, added and deleted files compared to the currently checked-out commit.
Show changed files which are not yet added for commit:
git status
Give output in [s]hort format:
git status --short
Show the [b]ranch and tracking info:
git status --branch
Show output in [s]hort format along with [b]ranch info:
git status --short --branch
Show the number of entries currently stashed away:
git status --show-stash
Don’t show untracked files in the output:
git status --untracked-files=no
git check-ref-format π
Check if a reference name is acceptable, and exit with a non-zero status if it is not.
Check the format of the specified reference name:
git check-ref-format refs/head/refname
Print the name of the last branch checked out:
git check-ref-format --branch @{-1}
Normalize a refname:
git check-ref-format --normalize refs/head/refname
git request-pull π
Generate a request asking the upstream project to pull changes into its tree.
Produce a request summarizing the changes between the v1.1 release and a specified branch:
git request-pull v1.1 <https://example.com/project> branch_name
Produce a request summarizing the changes between the v0.1 release on the foo
branch and the local bar
branch:
git request-pull v0.1 <https://example.com/project> foo:bar
git commit-tree π
Low level utility to create commit objects.
See also: git commit
.
Create a commit object with the specified message:
git commit-tree tree -m "message"
Create a commit object reading the message from a file (use -
for stdin
):
git commit-tree tree -F path/to/file
Create a GPG-signed commit object:
git commit-tree tree -m "message" --gpg-sign
Create a commit object with the specified parent commit object:
git commit-tree tree -m "message" -p parent_commit_sha
git unpack-file π
Create a temporary file with a blob’s contents.
Create a file holding the contents of the blob specified by its ID then print the name of the temporary file:
git unpack-file blob_id
git credential-cache π
Git helper to temporarily store passwords in memory.
Store Git credentials for a specific amount of time:
git config credential.helper 'cache --timeout=time_in_seconds'
git whatchanged π
Show what has changed with recent commits or files.
See also git log
.
Display logs and changes for recent commits:
git whatchanged
Display logs and changes for recent commits within the specified time frame:
git whatchanged --since="2 hours ago"
Display logs and changes for recent commits for specific files or directories:
git whatchanged path/to/file_or_directory
git mktree π
Build a tree object using ls-tree
formatted text.
Build a tree object and verify that each tree entryβs hash identifies an existing object:
git mktree
Allow missing objects:
git mktree --missing
Read the NUL ([z]ero character) terminated output of the tree object (ls-tree -z
):
git mktree -z
Allow the creation of multiple tree objects:
git mktree --batch
Sort and build a tree from stdin
(non-recursive git ls-tree
output format is required):
git mktree < path/to/tree.txt
git fetch π
Download objects and refs from a remote repository.
Fetch the latest changes from the default remote upstream repository (if set):
git fetch
Fetch new branches from a specific remote upstream repository:
git fetch remote_name
Fetch the latest changes from all remote upstream repositories:
git fetch --all
Also fetch tags from the remote upstream repository:
git fetch --tags
Delete local references to remote branches that have been deleted upstream:
git fetch --prune
git revert π
Create new commits which reverse the effect of earlier ones.
Revert the most recent commit:
git revert HEAD
Revert the 5th last commit:
git revert HEAD~4
Revert a specific commit:
git revert 0c01a9
Revert multiple commits:
git revert branch_name~5..branch_name~2
Don’t create new commits, just change the working tree:
git revert -n 0c01a9..9a1743
git ls-files π
Show information about files in the index and the working tree.
Show deleted files:
git ls-files --deleted
Show modified and deleted files:
git ls-files --modified
Show ignored and untracked files:
git ls-files --others
Show untracked files, not ignored:
git ls-files --others --exclude-standard
git for-each-repo π
Run a Git command on a list of repositories.
Note: this command is experimental and may change.
Run maintenance on each of a list of repositories stored in the maintenance.repo
user configuration variable:
git for-each-repo --config=maintenance.repo maintenance run
Run git pull
on each repository listed in a global configuration variable:
git for-each-repo --config=global_configuration_variable pull
git instaweb π
Helper to launch a GitWeb server.
Launch a GitWeb server for the current Git repository:
git instaweb --start
Listen only on localhost:
git instaweb --start --local
Listen on a specific port:
git instaweb --start --port 1234
Use a specified HTTP daemon:
git instaweb --start --httpd lighttpd|apache2|mongoose|plackup|webrick
Also auto-launch a web browser:
git instaweb --start --browser
Stop the currently running GitWeb server:
git instaweb --stop
Restart the currently running GitWeb server:
git instaweb --restart
git gui π
A GUI for Git to manage branches, commits, and remotes, and perform local merges.
Launch the GUI:
git gui
Show a specific file with author name and commit hash on each line:
git gui blame path/to/file
Open git gui blame
in a specific revision:
git gui blame revision path/to/file
Open git gui blame
and scroll the view to center on a specific line:
git gui blame --line=line path/to/file
Open a window to make one commit and return to the shell when it is complete:
git gui citool
Open git gui citool
in the “Amend Last Commit” mode:
git gui citool --amend
Open git gui citool
in a read-only mode:
git gui citool --nocommit
Show a browser for the tree of a specific branch, opening the blame tool when clicking on the files:
git gui browser maint
git verify-commit π
Check for GPG verification of commits.
If no commits are verified, nothing will be printed, regardless of options specified.
Check commits for a GPG signature:
git verify-commit commit_hash1 optional_commit_hash2 ...
Check commits for a GPG signature and show details of each commit:
git verify-commit commit_hash1 optional_commit_hash2 ... --verbose
Check commits for a GPG signature and print the raw details:
git verify-commit commit_hash1 optional_commit_hash2 ... --raw
git feature π
Create or merge feature branches.
Feature branches obey the format feature/<name>
.
Create and switch to a new feature branch:
git feature feature_branch
Merge a feature branch into the current branch creating a merge commit:
git feature finish feature_branch
Merge a feature branch into the current branch squashing the changes into one commit:
git feature finish --squash feature_branch
Send changes from a specific feature branch to its remote counterpart:
git feature feature_branch --remote remote_name
git tag π
Create, list, delete or verify tags.
A tag is a static reference to a commit.
List all tags:
git tag
Create a tag with the given name pointing to the current commit:
git tag tag_name
Create a tag with the given name pointing to a given commit:
git tag tag_name commit
Create an annotated tag with the given message:
git tag tag_name -m tag_message
Delete the tag with the given name:
git tag -d tag_name
Get updated tags from upstream:
git fetch --tags
List all tags whose ancestors include a given commit:
git tag --contains commit
git merge π
Merge branches.
Merge a branch into your current branch:
git merge branch_name
Edit the merge message:
git merge --edit branch_name
Merge a branch and create a merge commit:
git merge --no-ff branch_name
Abort a merge in case of conflicts:
git merge --abort
Merge using a specific strategy:
git merge --strategy strategy --strategy-option strategy_option branch_name
git merge-base π
Find a common ancestor of two commits.
Print the best common ancestor of two commits:
git merge-base commit_1 commit_2
Print all best common ancestors of two commits:
git merge-base --all commit_1 commit_2
Check if a commit is an ancestor of a specific commit:
git merge-base --is-ancestor ancestor_commit commit
git credential π
Retrieve and store user credentials.
Display credential information, retrieving the username and password from configuration files:
echo "url=<http://example.com>" | git credential fill
Send credential information to all configured credential helpers to store for later use:
echo "url=<http://example.com>" | git credential approve
Erase the specified credential information from all the configured credential helpers:
echo "url=<http://example.com>" | git credential reject
git show-merged-branches π
Print all branches which are merged into the current head.
Print all branches which are merged into the current head:
git show-merged-branches
git rm π
Remove files from repository index and local filesystem.
Remove file from repository index and filesystem:
git rm path/to/file
Remove directory:
git rm -r path/to/directory
Remove file from repository index but keep it untouched locally:
git rm --cached path/to/file
git checkout-index π
Copy files from the index to the working tree.
Restore any files deleted since the last commit:
git checkout-index --all
Restore any files deleted or changed since the last commit:
git checkout-index --all --force
Restore any files changed since the last commit, ignoring any files that were deleted:
git checkout-index --all --force --no-create
Export a copy of the entire tree at the last commit to the specified directory (the trailing slash is important):
git checkout-index --all --force --prefix=path/to/export_directory/
git check-mailmap π
Show canonical names and email addresses of contacts.
Look up the canonical name associated with an email address:
git check-mailmap "<[email protected]>"
git-grep π
Find strings inside files anywhere in a repository’s history.
Accepts a lot of the same flags as regular grep
.
Search for a string in tracked files:
git grep search_string
Search for a string in files matching a pattern in tracked files:
git grep search_string -- file_glob_pattern
Search for a string in tracked files, including submodules:
git grep --recurse-submodules search_string
Search for a string at a specific point in history:
git grep search_string HEAD~2
Search for a string across all branches:
git grep search_string $(git rev-list --all)
git ls-remote π
Git command for listing references in a remote repository based on name or URL.
If no name or URL are given, then the configured upstream branch will be used, or remote origin if the former is not configured.
Show all references in the default remote repository:
git ls-remote
Show only heads references in the default remote repository:
git ls-remote --heads
Show only tags references in the default remote repository:
git ls-remote --tags
Show all references from a remote repository based on name or URL:
git ls-remote repository_url
Show references from a remote repository filtered by a pattern:
git ls-remote repository_name "pattern"
git clean π
Remove files not tracked by Git from the working tree.
Delete untracked files:
git clean
[i]nteractively delete untracked files:
git clean -i
Show which files would be deleted without actually deleting them:
git clean --dry-run
[f]orcefully delete untracked files:
git clean -f
[f]orcefully delete untracked [d]irectories:
git clean -fd
Delete untracked files, including e[x]cluded files (files ignored in .gitignore
and .git/info/exclude
):
git clean -x
git am π
Apply patch files and create a commit. Useful when receiving commits via email.
See also git format-patch
, which can generate patch files.
Apply and commit changes following a local patch file:
git am path/to/file.patch
Apply and commit changes following a remote patch file:
curl -L <https://example.com/file.patch> | git apply
Abort the process of applying a patch file:
git am --abort
Apply as much of a patch file as possible, saving failed hunks to reject files:
git am --reject path/to/file.patch
git verify-tag π
Check for GPG verification of tags.
If a tag wasn’t signed, an error will occur.
Check tags for a GPG signature:
git verify-tag tag1 optional_tag2 ...
Check tags for a GPG signature and show details for each tag:
git verify-tag tag1 optional_tag2 ... --verbose
Check tags for a GPG signature and print the raw details:
git verify-tag tag1 optional_tag2 ... --raw
git format-patch π
Prepare .patch files. Useful when emailing commits elsewhere.
Create an auto-named .patch
file for all the unpushed commits:
git format-patch origin
Write a .patch
file for all the commits between 2 revisions to stdout
:
git format-patch revision_1..revision_2
Write a .patch
file for the 3 latest commits:
git format-patch -3
git mergetool π
Run merge conflict resolution tools to resolve merge conflicts.
Launch the default merge tool to resolve conflicts:
git mergetool
List valid merge tools:
git mergetool --tool-help
Launch the merge tool identified by a name:
git mergetool --tool tool_name
Don’t prompt before each invocation of the merge tool:
git mergetool --no-prompt
Explicitly use the GUI merge tool (see the merge.guitool
configuration variable):
git mergetool --gui
Explicitly use the regular merge tool (see the merge.tool
configuration variable):
git mergetool --no-gui
git show-ref π
Git command for listing references.
Show all refs in the repository:
git show-ref
Show only heads references:
git show-ref --heads
Show only tags references:
git show-ref --tags
Verify that a given reference exists:
git show-ref --verify path/to/ref
git diff-tree π
Compares the content and mode of blobs found via two tree objects.
Compare two tree objects:
git diff-tree tree-ish1 tree-ish2
Show changes between two specific commits:
git diff-tree -r commit1 commit2
Display changes in patch format:
git diff-tree -p tree-ish1 tree-ish2
Filter changes by a specific path:
git diff-tree tree-ish1 tree-ish2 -- path/to/file_or_directory
git show-branch π
Show branches and their commits.
Show a summary of the latest commit on a branch:
git show-branch branch_name|ref|commit
Compare commits in the history of multiple commits or branches:
git show-branch branch_name1|ref1|commit1 branch_name2|ref2|commit2 ...
Compare all remote tracking branches:
git show-branch --remotes
Compare both local and remote tracking branches:
git show-branch --all
List the latest commits in all branches:
git show-branch --all --list
Compare a given branch with the current branch:
git show-branch --current commit|branch_name|ref
Display the commit name instead of the relative name:
git show-branch --sha1-name --current current|branch_name|ref
Keep going a given number of commits past the common ancestor:
git show-branch --more 5 commit|branch_name|ref commit|branch_name|ref ...
git check-attr π
For every pathname, list if each attribute is unspecified, set, or unset as a gitattribute on that pathname.
Check the values of all attributes on a file:
git check-attr --all path/to/file
Check the value of a specific attribute on a file:
git check-attr attribute path/to/file
Check the values of all attributes on specific files:
git check-attr --all path/to/file1 path/to/file2 ...
Check the value of a specific attribute on one or more files:
git check-attr attribute path/to/file1 path/to/file2 ...
git ignore-io π
Generate .gitignore
files from predefined templates.
List available templates:
git ignore-io list
Generate a .gitignore template:
git ignore-io item_a,item_b,item_n
git mv π
Move or rename files and update the Git index.
Move a file inside the repo and add the movement to the next commit:
git mv path/to/file new/path/to/file
Rename a file or directory and add the renaming to the next commit:
git mv path/to/file_or_directory path/to/destination
Overwrite the file or directory in the target path if it exists:
git mv --force path/to/file_or_directory path/to/destination
git show-index π
Show the packed archive index of a Git repository.
Read an IDX file for a Git packfile and dump its contents to stdout
:
git show-index path/to/file.idx
Specify the hash algorithm for the index file (experimental):
git show-index --object-format=sha1|sha256 path/to/file
git write-tree π
Low level utility to create a tree object from the current index.
Create a tree object from the current index:
git write-tree
Create a tree object without checking whether objects referenced by the directory exist in the object database:
git write-tree --missing-ok
Create a tree object that represents a subdirectory (used to write the tree object for a subproject in the named subdirectory):
git write-tree --prefix subdirectory/
git remote π
Manage set of tracked repositories (“remotes”).
List existing remotes with their names and URLs:
git remote -v
Show information about a remote:
git remote show remote_name
Add a remote:
git remote add remote_name remote_url
Change the URL of a remote (use --add
to keep the existing URL):
git remote set-url remote_name new_url
Show the URL of a remote:
git remote get-url remote_name
Remove a remote:
git remote remove remote_name
Rename a remote:
git remote rename old_name new_name
git stage π
This command is an alias of git add .
git cat-file π
Provide content or type and size information for Git repository objects.
Get the [s]ize of the HEAD commit in bytes:
git cat-file -s HEAD
Get the [t]ype (blob, tree, commit, tag) of a given Git object:
git cat-file -t 8c442dc3
Pretty-[p]rint the contents of a given Git object based on its type:
git cat-file -p HEAD~2
git describe π
Give an object a human-readable name based on an available ref.
Create a unique name for the current commit (the name contains the most recent annotated tag, the number of additional commits, and the abbreviated commit hash):
git describe
Create a name with 4 digits for the abbreviated commit hash:
git describe --abbrev=4
Generate a name with the tag reference path:
git describe --all
Describe a Git tag:
git describe v1.0.0
Create a name for the last commit of a given branch:
git describe branch_name
git add π
Adds changed files to the index.
Add a file to the index:
git add path/to/file
Add all files (tracked and untracked):
git add -A
Only add already tracked files:
git add -u
Also add ignored files:
git add -f
Interactively stage parts of files:
git add -p
Interactively stage parts of a given file:
git add -p path/to/file
Interactively stage a file:
git add -i
git diff π
Show changes to tracked files.
Show unstaged changes:
git diff
Show all uncommitted changes (including staged ones):
git diff HEAD
Show only staged (added, but not yet committed) changes:
git diff --staged
Show changes from all commits since a given date/time (a date expression, e.g. “1 week 2 days” or an ISO date):
git diff 'HEAD@{3 months|weeks|days|hours|seconds ago}'
Show only names of changed files since a given commit:
git diff --name-only commit
Output a summary of file creations, renames and mode changes since a given commit:
git diff --summary commit
Compare a single file between two branches or commits:
git diff branch_1..branch_2 [--] path/to/file
Compare different files from the current branch to other branch:
git diff branch:path/to/file2 path/to/file
git archive π
Create an archive of files from a named tree.
Create a tar archive from the contents of the current HEAD and print it to stdout
:
git archive --verbose HEAD
Create a zip archive from the current HEAD and print it to stdout
:
git archive --verbose --format zip HEAD
Same as above, but write the zip archive to file:
git archive --verbose --output path/to/file.zip HEAD
Create a tar archive from the contents of the latest commit on a specific branch:
git archive --output path/to/file.tar branch_name
Create a tar archive from the contents of a specific directory:
git archive --output path/to/file.tar HEAD:path/to/directory
Prepend a path to each file to archive it inside a specific directory:
git archive --output path/to/file.tar --prefix path/to/prepend/ HEAD
git log π
Show a history of commits.
Show the sequence of commits starting from the current one, in reverse chronological order of the Git repository in the current working directory:
git log
Show the history of a particular file or directory, including differences:
git log -p path/to/file_or_directory
Show an overview of which file(s) changed in each commit:
git log --stat
Show a graph of commits in the current branch using only the first line of each commit message:
git log --oneline --graph
Show a graph of all commits, tags and branches in the entire repo:
git log --oneline --decorate --all --graph
Show only commits whose messages include a given string (case-insensitively):
git log -i --grep search_string
Show the last N commits from a certain author:
git log -n number --author=author
Show commits between two dates (yyyy-mm-dd):
git log --before="2017-01-29" --after="2017-01-17"
git svn π
Bidirectional operation between a Subversion repository and Git.
Clone an SVN repository:
git svn clone <https://example.com/subversion_repo> local_dir
Clone an SVN repository starting at a given revision number:
git svn clone -r1234:HEAD <https://svn.example.net/subversion/repo> local_dir
Update local clone from the remote SVN repository:
git svn rebase
Fetch updates from the remote SVN repository without changing the Git HEAD:
git svn fetch
Commit back to the SVN repository:
git svn commit
git commit π
Commit files to the repository.
Commit staged files to the repository with a message:
git commit --message "message"
Commit staged files with a message read from a file:
git commit --file path/to/commit_message_file
Auto stage all modified and deleted files and commit with a message:
git commit --all --message "message"
Commit staged files and sign them with the specified GPG key (or the one defined in the configuration file if no argument is specified):
git commit --gpg-sign key_id --message "message"
Update the last commit by adding the currently staged changes, changing the commit’s hash:
git commit --amend
Commit only specific (already staged) files:
git commit path/to/file1 path/to/file2
Create a commit, even if there are no staged files:
git commit --message "message" --allow-empty
git cherry π
Find commits that have yet to be applied upstream.
Show commits (and their messages) with equivalent commits upstream:
git cherry -v
Specify a different upstream and topic branch:
git cherry origin topic
Limit commits to those within a given limit:
git cherry origin topic base
git branch π
Main Git command for working with branches.
List all branches (local and remote; the current branch is highlighted by *
):
git branch --all
List which branches include a specific Git commit in their history:
git branch --all --contains commit_hash
Show the name of the current branch:
git branch --show-current
Create new branch based on the current commit:
git branch branch_name
Create new branch based on a specific commit:
git branch branch_name commit_hash
Rename a branch (must not have it checked out to do this):
git branch -m old_branch_name new_branch_name
Delete a local branch (must not have it checked out to do this):
git branch -d branch_name
Delete a remote branch:
git push remote_name --delete remote_branch_name
git clone π
Clone an existing repository.
Clone an existing repository into a new directory (the default directory is the repository name):
git clone remote_repository_location path/to/directory
Clone an existing repository and its submodules:
git clone --recursive remote_repository_location
Clone only the .git
directory of an existing repository:
git clone --no-checkout remote_repository_location
Clone a local repository:
git clone --local path/to/local/repository
Clone quietly:
git clone --quiet remote_repository_location
Clone an existing repository only fetching the 10 most recent commits on the default branch (useful to save time):
git clone --depth 10 remote_repository_location
Clone an existing repository only fetching a specific branch:
git clone --branch name --single-branch remote_repository_location
Clone an existing repository using a specific SSH command:
git clone --config core.sshCommand="ssh -i path/to/private_ssh_key" remote_repository_location
git check-ignore π
Analyze and debug Git ignore/exclude (".gitignore") files.
Check whether a file or directory is ignored:
git check-ignore path/to/file_or_directory
Check whether multiple files or directories are ignored:
git check-ignore path/to/file_or_directory1 path/to/file_or_directory2 ...
Use pathnames, one per line, from stdin
:
git check-ignore --stdin < path/to/file_list
Do not check the index (used to debug why paths were tracked and not ignored):
git check-ignore --no-index path/to/file_or_directory1 path/to/file_or_directory2 ...
Include details about the matching pattern for each path:
git check-ignore --verbose path/to/file_or_directory1 path/to/file_or_directory2 ...
git apply π
Apply a patch to files and/or to the index without creating a commit.
See also git am
, which applies a patch and also creates a commit.
Print messages about the patched files:
git apply --verbose path/to/file
Apply and add the patched files to the index:
git apply --index path/to/file
Apply a remote patch file:
curl -L <https://example.com/file.patch> | git apply
Output diffstat for the input and apply the patch:
git apply --stat --apply path/to/file
Apply the patch in reverse:
git apply --reverse path/to/file
Store the patch result in the index without modifying the working tree:
git apply --cache path/to/file
git switch π
Switch between Git branches. Requires Git version 2.23+.
See also git checkout .
Switch to an existing branch:
git switch branch_name
Create a new branch and switch to it:
git switch --create branch_name
Create a new branch based on an existing commit and switch to it:
git switch --create branch_name commit
Switch to the previous branch:
git switch -
Switch to a branch and update all submodules to match:
git switch --recurse-submodules branch_name
Switch to a branch and automatically merge the current branch and any uncommitted changes into it:
git switch --merge branch_name
git restore π
Restore working tree files. Requires Git version 2.23+.
See also git checkout and git reset .
Restore an unstaged file to the version of the current commit (HEAD):
git restore path/to/file
Restore an unstaged file to the version of a specific commit:
git restore --source commit path/to/file
Discard all unstaged changes to tracked files:
git restore :/
Unstage a file:
git restore --staged path/to/file
Unstage all files:
git restore --staged :/
Discard all changes to files, both staged and unstaged:
git restore --worktree --staged :/
Interactively select sections of files to restore:
git restore --patch
git rebase π
Reapply commits from one branch on top of another branch.
Commonly used to “move” an entire branch to another base, creating copies of the commits in the new location.
Rebase the current branch on top of another specified branch:
git rebase new_base_branch
Start an interactive rebase, which allows the commits to be reordered, omitted, combined or modified:
git rebase -i target_base_branch_or_commit_hash
Continue a rebase that was interrupted by a merge failure, after editing conflicting files:
git rebase --continue
Continue a rebase that was paused due to merge conflicts, by skipping the conflicted commit:
git rebase --skip
Abort a rebase in progress (e.g. if it is interrupted by a merge conflict):
git rebase --abort
Move part of the current branch onto a new base, providing the old base to start from:
git rebase --onto new_base old_base
Reapply the last 5 commits in-place, stopping to allow them to be reordered, omitted, combined or modified:
git rebase -i HEAD~5
Auto-resolve any conflicts by favoring the working branch version (theirs
keyword has reversed meaning in this case):
git rebase -X theirs branch_name
git cvsexportcommit π
Export a single Git
commit to a CVS checkout.
Merge a specific patch into CVS:
git cvsexportcommit -v -c -w path/to/project_cvs_checkout commit_sha1
git push π
Push commits to a remote repository.
Send local changes in the current branch to its default remote counterpart:
git push
Send changes from a specific local branch to its remote counterpart:
git push remote_name local_branch
Send changes from a specific local branch to its remote counterpart, and set the remote one as the default push/pull target of the local one:
git push -u remote_name local_branch
Send changes from a specific local branch to a specific remote branch:
git push remote_name local_branch:remote_branch
Send changes on all local branches to their counterparts in a given remote repository:
git push --all remote_name
Delete a branch in a remote repository:
git push remote_name --delete remote_branch
Remove remote branches that don’t have a local counterpart:
git push --prune remote_name
Publish tags that aren’t yet in the remote repository:
git push --tags
git bundle π
Package objects and references into an archive.
Create a bundle file that contains all objects and references of a specific branch:
git bundle create path/to/file.bundle branch_name
Create a bundle file of all branches:
git bundle create path/to/file.bundle --all
Create a bundle file of the last 5 commits of the current branch:
git bundle create path/to/file.bundle -5 HEAD
Create a bundle file of the latest 7 days:
git bundle create path/to/file.bundle --since=7.days HEAD
Verify that a bundle file is valid and can be applied to the current repository:
git bundle verify path/to/file.bundle
Print to stdout
the list of references contained in a bundle:
git bundle unbundle path/to/file.bundle
Unbundle a specific branch from a bundle file into the current repository:
git pull path/to/file.bundle branch_name
git lfs π
Work with large files in Git repositories.
Initialize Git LFS:
git lfs install
Track files that match a glob:
git lfs track '*.bin'
Change the Git LFS endpoint URL (useful if the LFS server is separate from the Git server):
git config -f .lfsconfig lfs.url lfs_endpoint_url
List tracked patterns:
git lfs track
List tracked files that have been committed:
git lfs ls-files
Push all Git LFS objects to the remote server (useful if errors are encountered):
git lfs push --all remote_name branch_name
Fetch all Git LFS objects:
git lfs fetch
Checkout all Git LFS objects:
git lfs checkout
git stripspace π
Read text (e.g. commit messages, notes, tags, and branch descriptions) from stdin
and clean it into the manner used by Git.
Trim whitespace from a file:
cat path/to/file | git stripspace
Trim whitespace and Git comments from a file:
cat path/to/file | git stripspace --strip-comments
Convert all lines in a file into Git comments:
git stripspace --comment-lines < path/to/file
git replace π
Create, list, and delete refs to replace objects.
Replace any commit with a different one, leaving other commits unchanged:
git replace object replacement
Delete existing replace refs for the given objects:
git replace --delete object
Edit an objectβs content interactively:
git replace --edit object
git hash-object π
Computes the unique hash key of content and optionally creates an object with specified type.
Compute the object ID without storing it:
git hash-object path/to/file
Compute the object ID and store it in the Git database:
git hash-object -w path/to/file
Compute the object ID specifying the object type:
git hash-object -t blob|commit|tag|tree path/to/file
Compute the object ID from stdin
:
cat path/to/file | git hash-object --stdin
gitk π
A graphical Git repository browser.
Show the repository browser for the current Git repository:
gitk
Show repository browser for a specific file or directory:
gitk path/to/file_or_directory
Show commits made since 1 week ago:
gitk --since="1 week ago"
Show commits older than 1/1/2016:
gitk --until="1/1/2015"
Show at most 100 changes in all branches:
gitk --max-count=100 --all
git reflog π
Show a log of changes to local references like HEAD, branches or tags.
Show the reflog for HEAD:
git reflog
Show the reflog for a given branch:
git reflog branch_name
Show only the 5 latest entries in the reflog:
git reflog -n 5
git blame π
Show commit hash and last author on each line of a file.
Print file with author name and commit hash on each line:
git blame path/to/file
Print file with author email and commit hash on each line:
git blame -e path/to/file
Print file with author name and commit hash on each line at a specific commit:
git blame commit path/to/file
Print file with author name and commit hash on each line before a specific commit:
git blame commit~ path/to/file
git init π
Initializes a new local Git repository.
Initialize a new local repository:
git init
Initialize a repository with the specified name for the initial branch:
git init --initial-branch=branch_name
Initialize a repository using SHA256 for object hashes (requires Git version 2.29+):
git init --object-format=sha256
Initialize a barebones repository, suitable for use as a remote over SSH:
git init --bare
git mailinfo π
Extract patch and authorship information from a single email message.
Extract the patch and author data from an email message:
git mailinfo message|patch
Extract but remove leading and trailing whitespace:
git mailinfo -k message|patch
Remove everything from the body before a scissors line (e.g. “–>* –”) and retrieve the message or patch:
git mailinfo --scissors message|patch
git-maintenance π
Run tasks to optimize Git repository data.
Register the current repository in the user’s list of repositories to daily have maintenance run:
git maintenance register
Start running maintenance on the current repository:
git maintenance start
Halt the background maintenance schedule for the current repository:
git maintenance stop
Remove the current repository from the user’s maintenance repository list:
git maintenance unregister
Run a specific maintenance task on the current repository:
git maintenance run --task=commit-graph|gc|incremental-repack|loose-objects|pack-refs|prefetch
git config π
Manage custom configuration options for Git repositories.
These configurations can be local (for the current repository) or global (for the current user).
List only local configuration entries (stored in .git/config
in the current repository):
git config --list --local
List only global configuration entries (stored in ~/.gitconfig
by default or in $XDG_CONFIG_HOME/git/config
if such a file exists):
git config --list --global
List only system configuration entries (stored in /etc/gitconfig
), and show their file location:
git config --list --system --show-origin
Get the value of a given configuration entry:
git config alias.unstage
Set the global value of a given configuration entry:
git config --global alias.unstage "reset HEAD --"
Revert a global configuration entry to its default value:
git config --global --unset alias.unstage
Edit the Git configuration for the current repository in the default editor:
git config --edit
Edit the global Git configuration in the default editor:
git config --global --edit
git help π
Display help information about Git.
Display help about a specific Git subcommand:
git help subcommand
Display help about a specific Git subcommand in a web browser:
git help --web subcommand
Display a list of all available Git subcommands:
git help --all
List the available guides:
git help --guide
List all possible configuration variables:
git help --config
git send-email π
Send a collection of patches as emails.
Patches can be specified as files, directions, or a revision list.
Send the last commit in the current branch:
git send-email -1
Send a given commit:
git send-email -1 commit
Send multiple (e.g. 10) commits in the current branch:
git send-email -10
Send an introductory email message for the patch series:
git send-email -number_of_commits --compose
Review and edit the email message for each patch you’re about to send:
git send-email -number_of_commits --annotate
git annotate π
Show commit hash and last author on each line of a file.
See git blame , which is preferred over git annotate .
git annotate
is provided for those familiar with other version control systems.
Print a file with the author name and commit hash prepended to each line:
git annotate path/to/file
Print a file with the author [e]mail and commit hash prepended to each line:
git annotate -e path/to/file
Print only rows that match a regular expression:
git annotate -L :regexp path/to/file
git fsck π
Verify the validity and connectivity of nodes in a Git repository index.
Does not make any modifications. git gc for cleaning up dangling blobs.
Check the current repository:
git fsck
List all tags found:
git fsck --tags
List all root nodes found:
git fsck --root
git prune π
Git command for pruning all unreachable objects from the object database.
This command is often not used directly but as an internal command that is used by Git gc.
Report what would be removed by Git prune without removing it:
git prune --dry-run
Prune unreachable objects and display what has been pruned to stdout
:
git prune --verbose
Prune unreachable objects while showing progress:
git prune --progress
git bisect π
Use binary search to find the commit that introduced a bug.
Git automatically jumps back and forth in the commit graph to progressively narrow down the faulty commit.
Start a bisect session on a commit range bounded by a known buggy commit, and a known clean (typically older) one:
git bisect start bad_commit good_commit
For each commit that git bisect
selects, mark it as “bad” or “good” after testing it for the issue:
git bisect good|bad
After git bisect
pinpoints the faulty commit, end the bisect session and return to the previous branch:
git bisect reset
Skip a commit during a bisect (e.g. one that fails the tests due to a different issue):
git bisect skip
Display a log of what has been done so far:
git bisect log
git update-index π
Git command for manipulating the index.
Pretend that a modified file is unchanged (git status
will not show this as changed):
git update-index --skip-worktree path/to/modified_file
Documentation & resources π
- Git: https://git-scm.com/ .
- Git bisect: https://git-scm.com/docs/git-bisect .
- Git prune: https://git-scm.com/docs/git-prune .
- Git lfs: https://git-lfs.github.com .
- Git ignore: https://github.com/tj/git-extras/blob/master/Commands.md#git-ignore-io .
- Git show-merged-branches: https://github.com/tj/git-extras/blob/master/Commands.md#git-show-merged-branches .
- Git feature: https://github.com/tj/git-extras/blob/master/Commands.md#git-feature .
- git submodule: https://git-scm.com/docs/git-submodule .
- git pull: https://git-scm.com/docs/git-pull .
- git reset: https://git-scm.com/docs/git-reset .
- git bugreport: https://git-scm.com/docs/git-bugreport .
- git gc: https://git-scm.com/docs/git-gc .
- git diff files: https://git-scm.com/docs/git-diff-files .
- git symbolic ref: https://git-scm.com/docs/git-symbolic-ref .
- git update ref: https://git-scm.com/docs/git-update-ref .
- git var: https://git-scm.com/docs/git-var .
- git range diff: https://git-scm.com/docs/git-range-diff .
- git stash: https://git-scm.com/docs/git-stash .
- git count objects: https://git-scm.com/docs/git-count-objects .
- git rev list: https://git-scm.com/docs/git-rev-list .
- git show: https://git-scm.com/docs/git-show .
- git checkout: https://git-scm.com/docs/git-checkout .
- git commit graph: https://git-scm.com/docs/git-commit-graph .
- git difftool: https://git-scm.com/docs/git-difftool .
- git rev parse: https://git-scm.com/docs/git-rev-parse .
- git repack: https://git-scm.com/docs/git-repack .
- git credential-store: https://git-scm.com/docs/git-credential-store .
- git daemon: https://git-scm.com/docs/git-daemon .
- git column: https://git-scm.com/docs/git-column .
- git cherry pick: https://git-scm.com/docs/git-cherry-pick .
- git worktree: https://git-scm.com/docs/git-worktree .
- git notes: https://git-scm.com/docs/git-notes .
- git ls tree: https://git-scm.com/docs/git-ls-tree .
- git shortlog: https://git-scm.com/docs/git-shortlog .
- git diff index: https://git-scm.com/docs/git-diff-index .
- git status: https://git-scm.com/docs/git-status .
- git check ref format: https://git-scm.com/docs/git-check-ref-format .
- git request pull: https://git-scm.com/docs/git-request-pull .
- git commit tree: https://git-scm.com/docs/git-commit-tree .
- git unpack file: https://git-scm.com/docs/git-unpack-file .
- git credential cache: https://git-scm.com/docs/git-credential-cache .
- git whatchanged: https://git-scm.com/docs/git-whatchanged .
- git mktree: https://git-scm.com/docs/git-mktree .
- git fetch: https://git-scm.com/docs/git-fetch .
- git revert: https://git-scm.com/docs/git-revert .
- git ls files: https://git-scm.com/docs/git-ls-files .
- git for each repo: https://git-scm.com/docs/git-for-each-repo .
- git instaweb: https://git-scm.com/docs/git-instaweb .
- git gui: https://git-scm.com/docs/git-gui .
- git verify-commit: https://git-scm.com/docs/git-verify-commit .
- git tag: https://git-scm.com/docs/git-tag .
- git merge: https://git-scm.com/docs/git-merge .
- git merge-base: https://git-scm.com/docs/git-merge-base .
- git credential: https://git-scm.com/docs/git-credential .
- git rm: https://git-scm.com/docs/git-rm .
- git checkout index: https://git-scm.com/docs/git-checkout-index .
- git check mailmap: https://git-scm.com/docs/git-check-mailmap .
- git grep: https://git-scm.com/docs/git-grep .
- git ls remote: https://git-scm.com/docs/git-ls-remote .
- git clean: https://git-scm.com/docs/git-clean .
- git am: https://git-scm.com/docs/git-am .
- git verify tag: https://git-scm.com/docs/git-verify-tag .
- git format patch: https://git-scm.com/docs/git-format-patch .
- git mergetool: https://git-scm.com/docs/git-mergetool .
- git show ref: https://git-scm.com/docs/git-show-ref .
- git diff tree: https://git-scm.com/docs/git-diff-tree .
- git show branch: https://git-scm.com/docs/git-show-branch .
- git check attr: https://git-scm.com/docs/git-check-attr .
- git mv: https://git-scm.com/docs/git-mv .
- git show index: https://git-scm.com/docs/git-show-index .
- git write tree: https://git-scm.com/docs/git-write-tree .
- git stage: https://git-scm.com/docs/git-stage .
- git cat file: https://git-scm.com/docs/git-cat-file .
- git describe: https://git-scm.com/docs/git-describe .
- git remote: https://git-scm.com/docs/git-remote .
- git add: https://git-scm.com/docs/git-add .
- git archive: https://git-scm.com/docs/git-archive .
- git log: https://git-scm.com/docs/git-log .
- git svn: https://git-scm.com/docs/git-svn .
- git commit: https://git-scm.com/docs/git-commit .
- git cherry: https://git-scm.com/docs/git-cherry .
- git branch: https://git-scm.com/docs/git-branch .
- git diff: https://git-scm.com/docs/git-diff .
- git clone: https://git-scm.com/docs/git-clone .
- git check ignore: https://git-scm.com/docs/git-check-ignore .
- git apply: https://git-scm.com/docs/git-apply .
- git switch: https://git-scm.com/docs/git-switch .
- git restore: https://git-scm.com/docs/git-restore .
- git rebase: https://git-scm.com/docs/git-rebase .
- git cvsexportcommit: https://git-scm.com/docs/git-cvsexportcommit .
- git push: https://git-scm.com/docs/git-push .
- git bundle: https://git-scm.com/docs/git-bundle .
- git stripspace: https://git-scm.com/docs/git-stripspace .
- git reflog: https://git-scm.com/docs/git-reflog .
- git replace: https://git-scm.com/docs/git-replace .
- git hash object: https://git-scm.com/docs/git-hash-object .
- gitk: https://git-scm.com/docs/gitk .
- git blame: https://git-scm.com/docs/git-blame .
- git init: https://git-scm.com/docs/git-init .
- git mailinfo: https://git-scm.com/docs/git-mailinfo .
- git maintenance: https://git-scm.com/docs/git-maintenance .
- git config: https://git-scm.com/docs/git-config .
- git help: https://git-scm.com/docs/git-help .
- git send-email: https://git-scm.com/docs/git-send-email .
- git annotate: https://git-scm.com/docs/git-annotate .
- git fsck: https://git-scm.com/docs/git-fsck .
- git update index: https://git-scm.com/docs/git-update-index .
I hope this post helps you. If you know a person who can benefit from this information, send them a link of this post. If you want to get notified about new posts, follow me on YouTube , Twitter (x) , LinkedIn , Facebook , and GitHub .
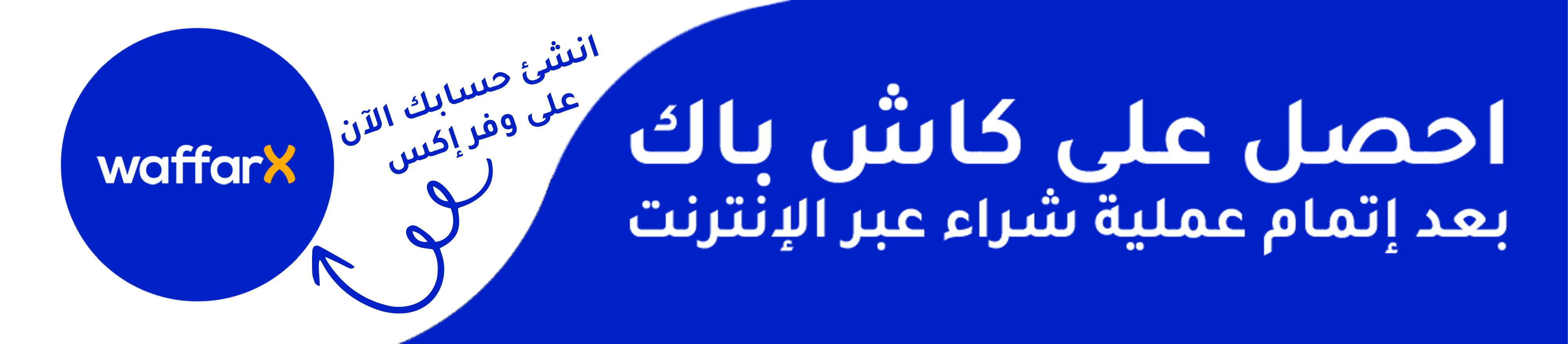