Unleash the Speed: Go Performance Optimization for Your Apps
Go, known for its simplicity and concurrency features, is a popular choice for building high-performance applications. But even the most elegant Go code can benefit from optimization techniques. This post explores performance optimization strategies in Go, drawing insights from practical examples.
Common Performance Bottlenecks ๐
- Inefficient Memory Usage: Unnecessary allocations, memory leaks, and improper data structures can significantly impact performance.
- Excessive Goroutines and Context Switching: While Goroutines are powerful for concurrency, managing too many can lead to context switching overhead.
- I/O Operations: Blocking I/O operations can stall your program’s execution.
Learning from the Cases ๐
Case 1: String Concatenation vs. String Builder ๐
This case demonstrates the performance difference between repeated string concatenation (bad) and using a StringBuilder (good). String concatenation creates a new string every time, leading to unnecessary memory allocations. StringBuilder offers a more efficient way to build strings incrementally.
The key takeaway is Favor memory-efficient data structures like StringBuilder for string manipulation to avoid repeated allocations.
Case 2: Optimizing a File Download Function ๐
This case study showcases the impact of I/O operations on performance. The original code downloads a file in a single blocking operation, hindering responsiveness. The optimized version uses buffered I/O and channels for non-blocking communication, improving efficiency.
Key Takeaways:
- Leverage non-blocking I/O operations like buffered channels to avoid blocking the main thread during I/O.
- Consider using concurrency models like worker pools to handle multiple downloads efficiently.
General Go Performance Optimization Tips ๐
- Profile your code: Use tools like pprof to identify performance bottlenecks.
- Optimize algorithms and data structures: Choose efficient algorithms and data structures suited to your tasks.
- Utilize concurrency effectively: Use Goroutines wisely and manage context switching overhead.
- Minimize unnecessary memory allocations: Reuse existing memory objects when possible.
- Handle errors efficiently: Avoid redundant error checks.
- Benchmark your code: Regularly benchmark your code to measure the impact of optimizations.
Remember: Optimization is not always about raw speed. It’s about striking a balance between performance, code readability, and maintainability.
By understanding common bottlenecks and applying optimization techniques, you can significantly improve the performance of your Go applications.
Read more about Go ๐
- Good Code vs Bad Code in Go
- Go code refactoring : the 23x performance hunt
- Don’t Communicate by Sharing Memory, Share Memory by Communicating: A Go Approach
I hope this post helps you. If you know a person who can benefit from this information, send them a link of this post. If you want to get notified about new posts, follow me on YouTube , Twitter (x) , LinkedIn , and GitHub .
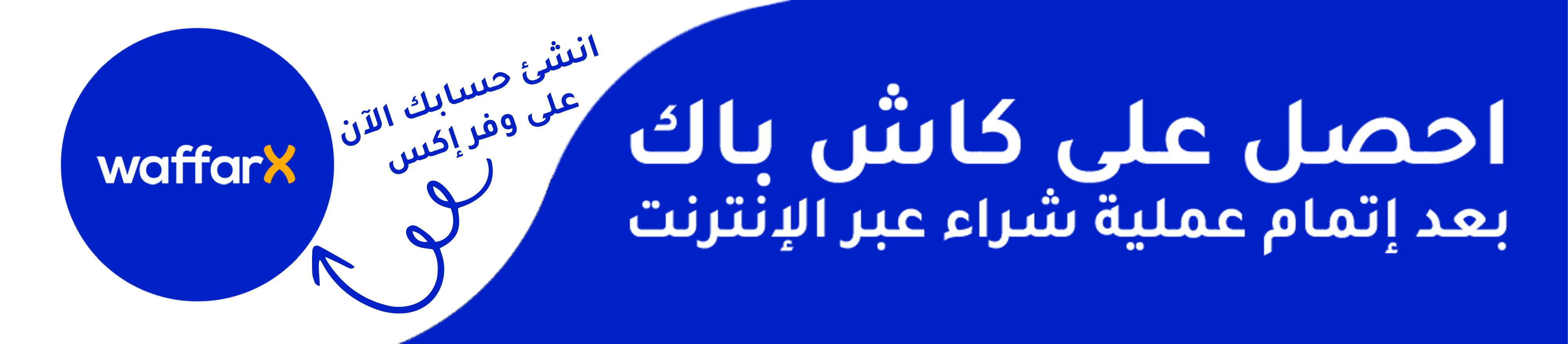