Go language | Tips & Tricks
I used Go language for almost 4 years now. I learned some tips and tricks dealing with Go projects.
Make the compiler count the array elements π
You donβt use arrays much in Go. Instead, you use slices, but when you do, if youβre tired of writing the number of elements in the array yourself, you can use [β¦]
and the compiler will count them for you:
package main
import "fmt"
func main() {
arr := [3]int{1, 2, 3}
sameArr := [...]int{1, 2, 3} // Use ... instead of 3
// Arrays are equivalent
fmt.Println(arr)
fmt.Println(sameArr)
}
Use go run .
instead of go run main.go
π
Whenever I start prototyping in Go, I like to start with a main.go file:
package main
import "fmt"
func main() {
sayHello()
}
func sayHello() {
fmt.Println("Hello!")
}
But when the main.go
file gets big, I like to split my structs into separate files using the same main
package. However, if I have the following files:
main.go
package main
func main() {
sayHello()
}
say_hello.go
package main
import "fmt"
func sayHello() {
fmt.Println("Hello!")
}
Typing go run main.go
gives the following error:
# command-line-arguments
./main.go:4:2: undefined: sayHello
To solve this, use go run .
Use the underscore to make your numbers readable π
Did you know that you can use the underscore to make your long numbers easier to read?
package main
import "fmt"
func main() {
number := 10000000
better := 10_000_000
fmt.Println(number == better)
}
Removing Debug Information for release builds π
By default, the Go compiler includes a symbol table and debug information in the compiled program. Typically, you can remove this debug information for a release version to reduce the binary size.
$ go build -o app main.go
$ ls -lh app
-rwxr-xr-x 1 ubuntu ubuntu 6.5M Jul 2 14:20 app
$ go build -ldflags="-s -w" -o app main.go
$ ls -lh app
-rwxr-xr-x 1 ubuntu ubuntu 4.5M Jul 2 14:30 app
-s
: Omit the symbol table and debug information.-w
: Omit the DWARFv3 debug information. With this option, you cannot use gdb to debug.
The size dropped from 6.5M to 4.5M, about a 30% reduction.
Compressed binary/executable π
UPX is an advanced executable file compressor. UPX will typically reduce the file size of programs and DLLs by around 50%-70%, thus reducing disk space, network load times, download times, and other distribution and storage costs.
On Mac, you can install UPX via brew .
brew install upx
- Compressing with UPX Alone
$ go build -o app main.go && upx --brute app && ls -lh app
-rwxr-xr-x 1 ubuntu ubuntu 3.9M Jul 2 14:38 app
6.5M -> 3.9M The compression ratio is about 60%.
- UPX + Compiler Options
Enabling both UPX and -ldflags="-s -w"
:
$ go build -ldflags="-s -w" -o app main.go && upx --brute app && ls -lh app
-rwxr-xr-x 1 ubuntu ubuntu 1.4M Jul 2 14:40 app
6.5MB -> 1.4MB saving about 80% of the space.
I hope you enjoyed reading this post as much as I enjoyed writing it. If you know a person who can benefit from this information, send them a link of this post. If you want to get notified about new posts, follow me on YouTube , Twitter (x) , LinkedIn , and GitHub .
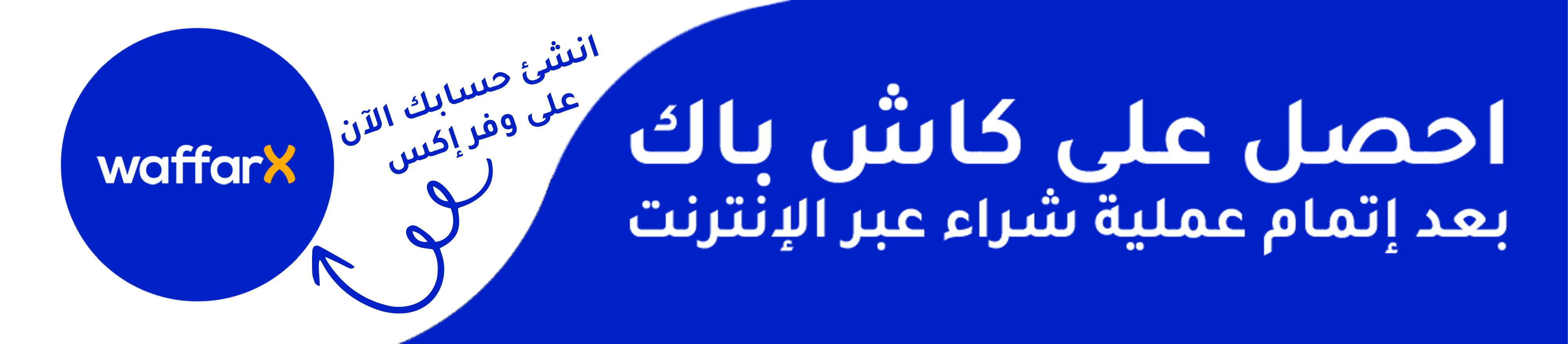