Laravel Commands Cheatsheet
laravel new project-name
๐
create a new folder named “project-name” in the current directory. Then create all necessary Laravel project files inside it.
composer require vendor/package
๐
update composer.json with the necessary details of the package you are choosing to install, then install the package in your project.
composer update
๐
update the installed packages.
composer dump-autoload
๐
updates your vendor/composer/autoload_classmap.php
file. You have to run this command if you have a new class in your project that has not yet been loaded.
php artisan list
๐
list all the artisan commands
php artisan --help
or php artisan -h
๐
display some basic help.That shows all/some the available flags for php artisan
php artisan key:generate
๐
generates a new key and adds it to your .env
file. A key is automatically generated and added to .env
file when you use laravel new projectName
. But it is useful when you clone an existing project. This app key is mainly ised for encrypting cookies
.
php artisan --version
or php artisan -V
๐
displays your current version of Laravel.
php artisan down
๐
puts your application into maintenance mode. Visitors will see the maintenance message.
php artisan up
๐
brings your application (website) back out of maintenance mode.
php artisan env
๐
displays the current environment for your application (website) like this Current application environment:local
php artisan route:list
๐
lists all the routes registered in your application, under the following headers:
Domain, Method, URI, Name, Action, Middelware
php artisan serve
๐
runs a local web server and tells you the local url to use to show the website / application. If you want to specify a port and host use a command like this php artisan serve --host=192.168.1.200 --port=80
.
php artisan make:auth
๐
creates all that is necessary for authentication in your application. Make sure you run php artisan migrate
after this command. After that, you can navigate to /register
or /login
on your project to create and log in an account.
php artisan make:model ModelName -mcr
๐
creates a model class and file in your project. You can use some, none or all of the -mcr
flags when creating a new model.
flag | meaning |
---|---|
-m or --migration | creates a new database migration file for the model |
-c or --controller | creates a new controller file for the model |
-r or --resources | indicates if the generates controller should be a resource controller |
If you want to see all options, run this command php artisan make:model -h
.
php artisan make:controller ControllerName
๐
creates a controller file in your project. You can specify a controller for which model via this flag --model=ModelName
so the command will be php artisan make:controller UserController --model=User
if the User
is the name of a model.
php artisan make:migration --table='table' 'description_of_migration'
๐
creates a database migration file that you can edit to add necessary table properties.
php artisan migrate
๐
runs any pending database migrations.
php artisan migrate:rollback
๐
Rolls back the latest database migration (ensuring you have the necessary commands in your down()
function of the migration).
if you want to roll back the last 5 migrations, just use this command php artisan migrate:rollback --step=5
.
php artisan migrate:reset
๐
rolls back all migrations.
php artisan vendor:publish
๐
displays a list of vendor packages installed in your project, giving you the option to specify which you would like to copy the configuration or view files toyour own project’s folders for additional configuration or customization.
php artisan route:cache
๐
speed up your application for production caching all your application’s routes.
php artisan route:clear
๐
clear the cached version of your routes โ use this on local deployments if you have cached routes. Re-run the cache command above on production to clear and re-cache routes.
php artisan clear-compiled
๐
remove the compiled class file.
php artisan optimize
๐
cache the framework bootstrap files.
php artisan test
๐
run the application tests.
php artisan tinker
๐
interact with your application.
php artisan auth:clear-resets
๐
flush expired password reset tokens
Cache ๐
php artisan cache:clear
๐
flush the application cache.
php artisan cache:forget
๐
remove an item from the cache.
php artisan cache:table
๐
create a migration for the cahce database table.
Config ๐
php artisan config:cache
๐
create a cache file for faster configuration loading. That speeds up your application for production by combining all your config options into a single file that loads quickly.
php artisan config:clear
๐
removes / clears your cached config (configuration cache file)โuse this on local deployments if you have cached config. Re-run the cache comman above on production to clear and re-cache config.
database (db) ๐
php artisan db:seed
๐
seed the database with records.
php artisan db:wipe
๐
delete / drop all tables, views, and types.
Event ๐
php artisan event:cache
๐
discover and cache the application’s events and listeners.
php artisan event:clear
๐
clear all cached events and listeners.
php artisan event:generate
๐
generate the missing events and listeners based on registration.
php artisan event:list
๐
list the application’s events and listeners.
make
commands ๐
php artisan command | meaning |
---|---|
make:channel | Create a new channel class |
make:command | Create a new Artisan command |
make:component | Create a new view component class |
make:controller | Create a new controller class |
make:event | Create a new event class |
make:exception | Create a new custom exception class |
make:factory | Create a new model factory |
make:job | Create a new job class |
make:listener | Create a new event listener class |
make:mail | Create a new email class |
make:middleware | Create a new middleware class |
make:migration | Create a new migration file |
make:model | Create a new Eloquent model class |
make:notification | Create a new notification class |
make:observer | Create a new observer class |
make:policy | Create a new policy class |
make:provider | Create a new service provider class |
make:request | Create a new form request class |
make:resource | Create a new resource |
make:rule | Create a new validation rule |
make:seeder | Create a new seeder class |
make:test | Create a new test class |
migrate
commands ๐
php artisan command | meaning |
---|---|
migrate:fresh | Drop all tables and re-run all migrations |
migrate:install | Create the migration repository |
migrate:refresh | Reset and re-run all migrations |
migrate:reset | Rollback all database migrations |
migrate:rollback | Rollback the last database migration |
migrate:status | Show the status of each migration |
php artisan notifications:table
๐
creates a migration for the notifications table.
php artisan optimize:clear
๐
Remove the cached bootstrap files
php artisan package:discover
๐
Rebuild the cached package manifest
queue commands ๐
php artisan command | meaning |
---|---|
queue:failed | List all of the failed queue jobs |
queue:failed-table | Create a migration for the failed queue jobs database table |
queue:flush | Flush all of the failed queue jobs |
queue:forget | Delete a failed queue job |
queue:listen | Listen to a given queue |
queue:restart | Restart queue worker daemons after their current job |
queue:retry | Retry a failed queue job |
queue:table | Create a migration for the queue jobs database table |
queue:work | Start processing jobs on the queue as a daemon |
route commands ๐
php artisan command | meaning |
---|---|
route:cache | Create a route cache file for faster route registration |
route:clear | Remove the route cache file |
route:list | List all registered routes |
php artisan schedule:run
๐
Run the scheduled commands.
php artisan session:table
๐
Create a migration for the session database table.
php artisan storage:link
๐
Create the symbolic links configured for the application.
php artisan stub:publish
๐
Publish all stubs that are available for customization.
view
commands ๐
command | meaning |
---|---|
php artisan view:cache | Compile all of the application’s Blade templates |
php artisan view:clear | Clear all compiled view files |
Final thoughts & tips ๐
I personally add an alias for php artisan
because I have to write it again and again. I just add this line to the end of my .zshrc
or .bashrc
on my Linux or Mac.
alias pa="php artisan"
So, I can write pa serve
which means php artisan serve
.
If you have any recommendations or tips or questions for me, tweet to me on twitter . And if you found this post useful, recommend it to a friend or share it with him/her.
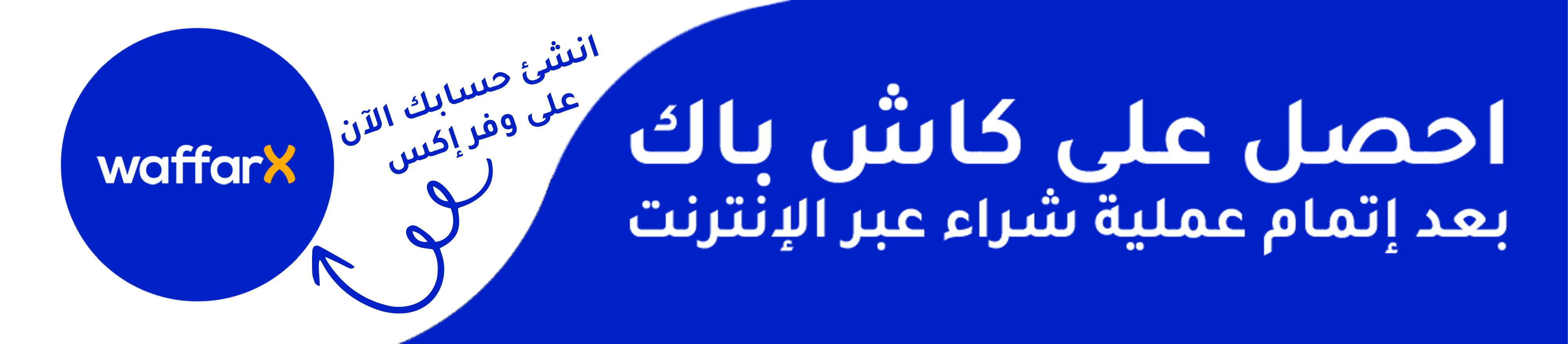