Make Your Flutter App Slim and Trim: Size Optimization Tips
Ever published a Flutter app and winced at the download size? Here are some tricks to make your app svelte and save users precious storage space.
Images π
Supercharge your Icons with SVG: Instead of clunky JPGs or PNGs, use Scalable Vector Graphics (SVG) for icons and vector images. SVG icons are much smaller and won’t lose quality when resized for different screens.
WEBP power: For photos, consider using WEBP instead of JPG or PNG. It offers similar image quality but at a smaller size (around 25% smaller!). Check out the guide from Google Developers for more info on WEBP: https://developers.google.com/speed/webp .
Unused Stuff? Shake it Out π
Flutter can remove unused icons from fonts like Material, Cupertino, and FontAwesome. This is called “tree shaking”.
Shake on iOS: Building from Xcode? Set the
TREE_SHAKE_ICONS
environment variable.Shake on Android: Add the
tree-shake-icons
property to yourgradle.properties
file.
Compression Magic π
We all know to compress JPGs and PNGs, but did you know SVG and WEBP images can be compressed too?
Zip your Animations: Compress those animations to save space!
Gzip Everything: Use gzip compression to shrink files like language files (JSON). The provided code snippet shows how to use gzip to compress a file. You can find more info on this technique here .
final String response = await rootBundle.loadString('assets/en_us.json');
List<int> original = utf8.encode(response);
List<int> compressed = gzip.encode(original);
List<int> decompress = gzip.decode(compressed);
final enUS = utf8.decode(decompress);
Android Specific Tips π
Proguard Power: Proguard helps shrink, obfuscate, and optimize your code. The guide includes steps to add Proguard rules to your
android/app/build.gradle
andandroid/app/proguard-rules.pro
files. See this article for more on Proguard.Add to the file
android/app/build.gradle
android { buildTypes { getByName("release") { // Enables code shrinking, obfuscation, and optimization minifyEnabled = true // Enables resource shrinking shrinkResources = true // Enables proguard rules proguardFiles( getDefaultProguardFile("proguard-android-optimize.txt")), "proguard-rules.pro" ) } } }
Add to the file
android/app/proguard-rules.pro
## Flutter wrapper -keep class io.flutter.app.** { *; } -keep class io.flutter.plugin.** { *; } -keep class io.flutter.util.** { *; } -keep class io.flutter.view.** { *; } -keep class io.flutter.** { *; } -keep class io.flutter.plugins.** { *; } -keep class com.google.firebase.** { *; } // uncomment this if you are using firebase in the project -dontwarn io.flutter.embedding.** -ignorewarnings
Obfuscate for Security and Size: Obfuscating your code makes it harder to understand and reduces its size. The guide includes links on how to enable obfuscation for Android and iOS.
For Android, add to this file
/android/gradle.properties
.extra-gen-snapshot-options=--obfuscate
For iOS, read this guide .
Google Fonts on Demand: Use the
google_fonts
package to dynamically download fonts only when they are used by your app. This saves space by avoiding unnecessary font variants. Check out the package on pub.dev: https://pub.dev/packages/google_fonts .Cache those Images: The
cached_network_image
package automatically caches downloaded images, saving space and bandwidth. Find it on pub.dev: https://pub.dev/packages/cached_network_image .Supercharge Optimizations: Enable more aggressive optimizations in your
android/gradle.properties
file to remove unused code and combine classes, resulting in a smaller app. By adding this lineandroid.enableR8.fullMode=true
. For more info, read this guide by AndroidDeveloper .Compress those Native Libraries: Reduce the size of native libraries to save space on devices. See the provided link for more info. Add this line
android.bundle.enableUncompressedNativeLibs=true
into the end of this fileandroid/gradle.properties
. Read more about this on StackOverflow .Split Debug Info: Splitting debug info from your code creates a smaller APK size. Use this command to achieve this
flutter build appbundle --split-debug-info=/<path-to-export>
.
Think Ahead: Deferred Components π
Flutter allows you to build apps that download additional features and assets at runtime. This reduces the initial download size and lets users get what they need as they go. Learn more about deferred components in the official Flutter documentation here .
Find the Bulky Bits π
Identify Heavy Packages: Use the flutter build
command with the --analyze-size
flag to identify large packages in your app. The guide shows specific commands for analyzing Android App Bundles, Android APKs, and iOS builds.
For Androidβs AppBundle:
flutter build appbundle --target-platform android-arm64 --analyze-size
For Androidβs APK:
flutter build apk --target-platform android-arm64 --analyze-size
For iOS:
flutter build ios --analyze-size
By following these tips, you can significantly reduce the size of your Flutter app, making it a joy to download and use
References & resources π
- Reduce apk size: https://developer.android.com/topic/performance/reduce-apk-size
- shrink code: https://developer.android.com/studio/build/shrink-code
- size matters! reducing Flutter app size: https://suryadevsingh24032000.medium.com/size-matters-reducing-flutter-app-size-best-practices-ca992207782
- reducing Flutter app size: https://itnext.io/reducing-flutter-app-size-570db9810ebb
- how to obfuscate Flutter apps: https://newbedev.com/how-to-obfuscate-flutter-apps
I hope this post helps you. If you know a person who can benefit from this information, send them a link of this post. If you want to get notified about new posts, follow me on YouTube , Twitter (x) , LinkedIn , Facebook , and GitHub .
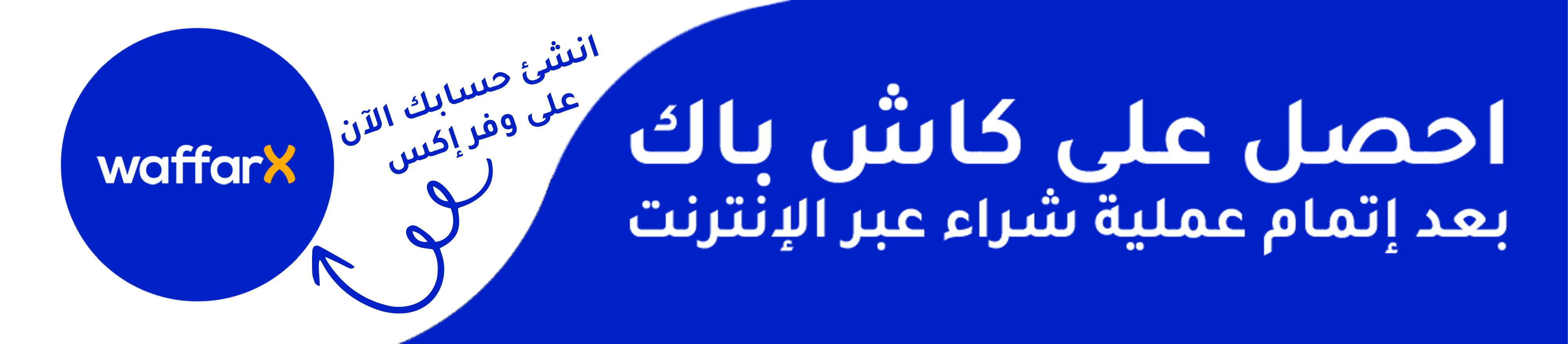