10 Tips to optimize Flutter mobile app
Use the Flutter Profiler to identify performance issues.
Use the Flutter DevTools to debug your app.
Reduce the number of widgets in your app and use lazy loading where possible.
Use the Dart compiler to optimize your code for faster execution.
Use the latest version of Flutter and its dependencies for better performance.
Avoid using large images and videos in your app, instead use smaller versions or thumbnails where possible.
Pre-compile your code for faster execution time and reduced memory usage.
Utilize caching techniques to reduce network requests and improve loading times for frequently accessed data or resources in your app.
Minimize the number of network requests by combining multiple requests into one request when possible, or by using batching techniques when appropriate
Utilize platform-specific features such as Androidβs JobScheduler or iOSβs Background Fetch to perform tasks in the background without draining battery life
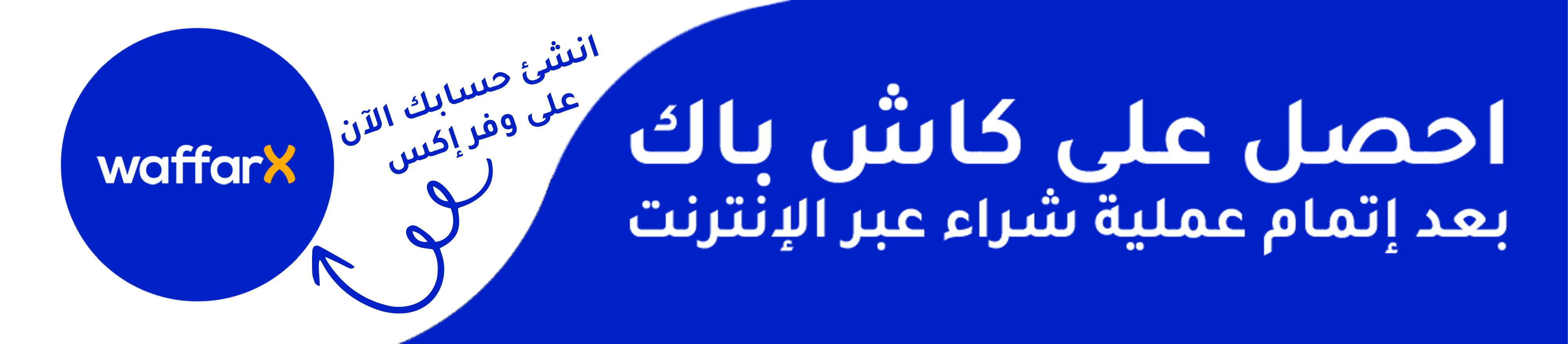